Actor#
Actor: Grundbausteine deiner Welt#
An actor is anything that can move or be changed in your world. It could be a character controlled by the player, or an object like a wall or an obstacle. In Miniworlds, every actor is an independent object that is displayed in the world and can interact with other objects.
Create an actor#
Fangen wir damit an, einen Akteur in deiner Welt zu platzieren. Zuerst erstellen wir eine einfache Welt mit einem Hintergrund und platzieren einen Akteur.
This goes like this:
import miniworlds
# Erstelle eine Welt mit den Maßen 600x300 Pixel
world = miniworlds.World(600, 300)
# Füge einen Hintergrund hinzu (zum Beispiel ein Grasbild)
world.add_background("images/grass.png")
# Erstelle einen Actor
actor = miniworlds.Actor((100, 40)) # Actor an der Position (0, 0)
# Starte die Welt, damit sie angezeigt wird
world.run()
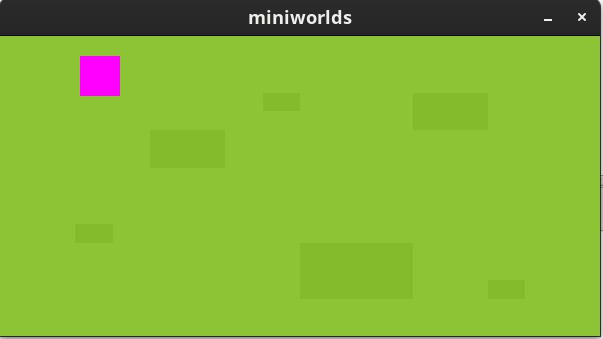
Ausgabe#
Explanation:#
We create an actor at position x=100, y=40. It will be displayed as a purple rectangle because it does not have a costume yet.
Note, dass der Ursprung des Koordinatensystems oben links liegt.
Example: The coordinate system#
The position (100, 20) refers to the center of the actor.
Kostüme#
Every actor in Miniworlds can wear a costume that determines their outward appearance.
A costume is simply an image that you assign to your actor to give it a visual identity.
Step 1: Prepare images#
Before you can add a costume to an actor, you must copy the corresponding images into the images folder of your project. A typical project structure might look like this:
project
│ my_world.py # file with your python code
└───images
│ │ grass.png
│ │ knight.png
│ │ player.png
Schritt 2: Kostüm hinzufügen#
Once you have prepared your images, you can assign an image as a costume to your actor using the add_costume()
method.
from miniworlds import World, Actor
# Erstelle eine Welt mit den Maßen 600x300
world = World(600, 300)
# Füge einen Hintergrund hinzu
world.add_background("images/grass.png")
# Erstelle den ersten Actor an der Position (100, 20) und füge ein Kostüm hinzu
actor2 = Actor((100, 20))
actor2.add_costume("images/knight.png") # "knight.png" als Kostüm
# Starte die Welt, damit die Actors sichtbar sind
world.run()
Output:#
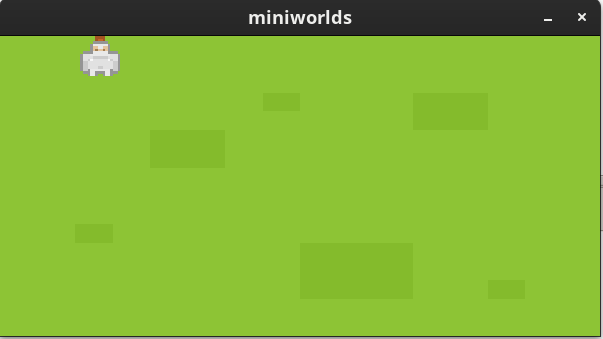
Ausgabe#
Erklärung#
After running, you will see an actor with the costume knight.png
.
Bonus: Experimentiere mit eigenen Kostümen!#
Now that you know how to assign costumes, you can get creative:
Create your own images and save them in the images folder.
Change the position and appearance of your actors.
Versuchen Sie beispielsweise, einen Actor an einer neuen Position zu erstellen und ihm ein anderes Bild zuzuweisen:
# Füge einen dritten Actor hinzu und gib ihm ein eigenes Kostüm
actor3 = Actor((200, 150))
actor3.add_costume("images/cow.png") # Kostüm: "cow.png"
# Starte die Welt erneut
world.run()
Zusammenfassung:#
Actors erhalten ein Kostüm durch die Methode add_costume().
The images must be saved in the correct folder to be found.
You can place and design any number of actors with different costumes in the world.
Häufig gestellte Fragen
FAQ: Häufige Probleme und Lösungen
My actor is misaligned, what can I do?
If your actor is facing the wrong direction, there are two simple solutions:
Problem description
Problem
from miniworlds import World, Actor
world = World()
world.add_background("images/grass.jpg")
player = Actor((90,90))
player.add_costume("images/player_orientation_top.png")
player.direction = "right"
world.run()

The orientation of the image is upwards. Miniworlds, however, expects images that are oriented to the right.#
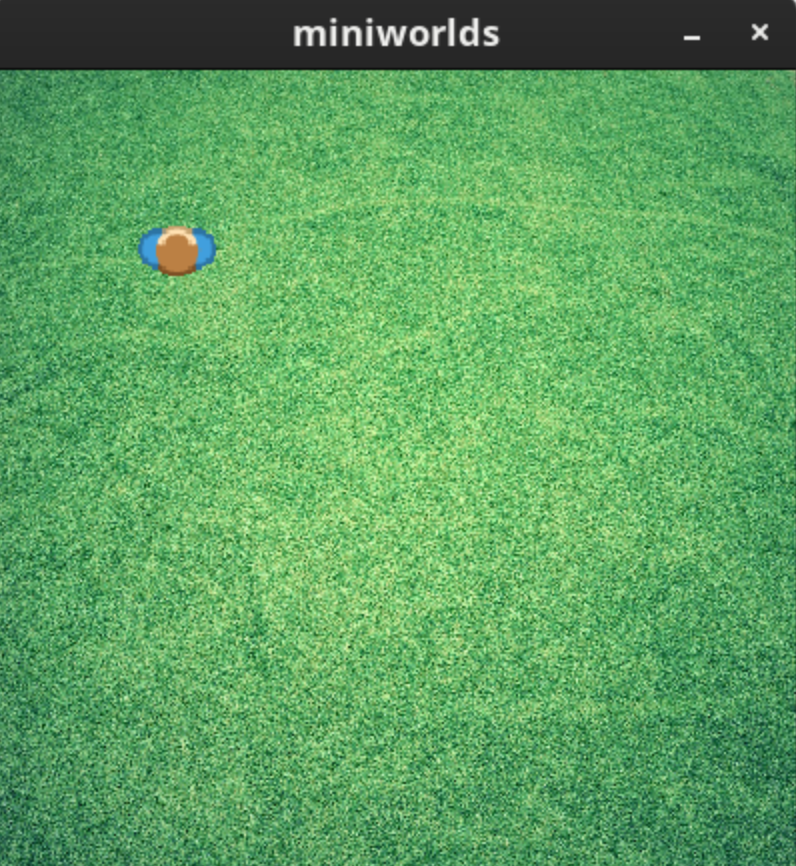
Therefore, the actor in the example is looking in the wrong direction#
Solution
Rotate image: You can rotate the image in an image editing program so that it points in the desired direction (usually upwards).
Orientierung im Code anpassen: Alternativ kannst du die Ausrichtung des Kostüms direkt in Miniworlds ändern. Verwende dazu das Attribut
orientation
, um das Kostüm zu drehen:my_actor.costume.orientation = 90 # Dreht das Kostüm um 90 Grad
You can also use other values like -90 or 180 to adjust the orientation, depending on how your original image is aligned.
The example above could be corrected as follows:
from miniworlds import World, Actor
world = World()
world.add_background("images/grass.jpg")
player = Actor((90,90))
player.add_costume("images/player_orientation_top.png")
player.direction = "right"
player.costume.orientation = -90
world.run()
Explanation:
The image is rotated -90° to the left from the expected position. With this additional information, the image is now correctly aligned.
How do I prevent the costume from rotating with the actor?
How do I prevent the costume from rotating with the actor?
If you want the costume not to rotate even when the actor moves or rotates, you can disable the rotation of the costume. To do this, set the attribute is_rotatable to False:
my_actor.costume.is_rotatable = False # Kostüm bleibt fest ausgerichtet