Concept: Schleifen#
Die for-Schleife#
Die for-Schleife wiederholt -vereinfacht gesprochen- einen Befehl n-mal:
Example#
The following loop will run 5 times:
for i in range(5):
print("I'm in a loop!")
The program produces the following output
I'm in a loop!
I'm in a loop!
I'm in a loop!
I'm in a loop!
I'm in a loop!
The counter variable#
You can use the variable i as a counter variable. It counts up (starting from 0):
Example#
for i in range(5):
print(i)
The program produces the following output
0
1
2
3
4
General form:#
In der Regel schreibt man:
for i in range(max):
<codeblock>
oder
for i in range(min, max):
<codeblock>
You can specify how often the loop is run or specify certain ranges:
Beispiele: Mit Schleifen zeichnen#
With loops, you can draw:
import miniworlds
world = miniworlds.World(200, 200)
for i in range(4):
miniworlds.Circle((20 + 50 * i, 50), 20)
world.run()
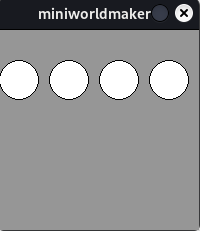
Schachbrettmuster#
With the modulo operator, you can check if a result is divisible by 2, namely ``x divisible by 2 if and only if x % 2 == 0`
This can be used to draw checkerboard patterns by combining loops with an if statement:
from miniworlds import *
world = World(200, 50)
for i in range(4):
rect = Rectangle((50 * i, 0), 50, 50)
if i % 2 == 0:
rect.color = (255,0,0, 255)
else:
rect.color = (255, 255, 255, 255)
world.run()
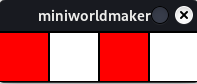
Graphen#
Auch Graphen lassen sich auf diese Art zeichnen:
from miniworlds import *
world = World(400, 400)
for x in range(400):
gl = 0.5*x + 50
y = 400 - gl
Point((x, y))
world.run()
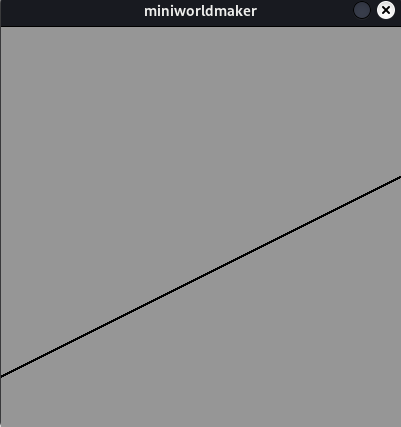
Nested loops#
With the help of nested loops, you can draw multidimensional patterns.
from miniworlds import *
world = World(200, 200)
for i in range(4):
for j in range(4):
Circle((20 + 50 * i, 20 + 50 * j), 20)
world.run()
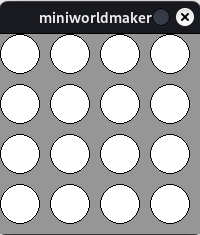
Die while-Schleife#
Die while-Schleife hat allgemein folgenden Aufbau:
while <Bedingung>:
<code-block>
As long as the condition is true, the loop will repeat over and over again. This also makes it possible to create infinite loops.
Example:
The following program generates a random pattern:
from miniworlds import *
import random
world = World(255, 60)
x = 0
while x < 255:
c = Circle((x, 30), 20)
c.color = (x,0,0,random.randint(0,255))
x = x + random.randint(10,50)
world.run()
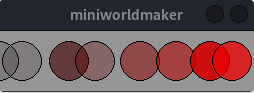
Die Hauptschleife#
Your entire program technically runs within a while loop:
while <no quit>
<draw images on screen>
<handle logic and events>
The for loop is not suitable for this, as you need to know in advance how many iterations should be executed.
Loops innerhalb von registrierten Methoden#
If you want to run a loop within the act
method or an event method, then you need to know the following:
The entire loop within such a method is executed within a single frame. Therefore, a loop is not suitable here for moving a character, for example, since it is redrawn every frame - However, a loop runs completely within a frame.
Example#
This can be well illustrated using the example of a traffic light system. The sequence of the loop can be well visualized with a state diagram.
stateDiagram [*] --> Green Green --> GreenYellow GreenYellow --> Red Red --> RedYellow RedYellow --> Green
In code, you can implement this as follows:
from miniworlds import *
state = "green"
while True:
if state == "green":
state = "green-yellow"
print("green")
elif state == "green-yellow":
state = "red"
print("green-yellow")
elif state == "red":
state = "red-yellow"
print("red")
elif state == "red-yellow":
state = "green"
print("red-yellow")
world.run()
In the main loop, the while loop is “replaced” by the main loop:
from miniworlds import *
world = World(100,240)
state = "green"
g = Circle ((50,40), 40)
y = Circle ((50,120), 40)
r = Circle ((50,200), 40)
@world.register
def act(self):
global state
if world.frame % 20 == 0:
if state == "green":
g.color = (0,255,0)
y.color = (255,255,255)
r.color = (255,255,255)
state = "green-yellow"
print("green")
elif state == "green-yellow":
g.color = (0,255,0)
y.color = (255,255,0)
r.color = (255,255,255)
state = "red"
print("green-yellow")
elif state == "red":
g.color = (255,255,255)
y.color = (255,255,255)
r.color = (255,0,0)
state = "red-yellow"
print("red")
elif state == "red-yellow":
g.color = (255,255,255)
y.color = (255,255,0)
r.color = (255,0,0)
state = "green"
print("red-yellow")
world.run()
As you can see, the program code has only been changed in certain places, the program flow remains the same. The while
loop is replaced here by the act
method.