Colors and Contours#
Colorieren#
A geometric shape can be colored with the attribute fill_color
:
from miniworlds import *
world = World(350, 150)
r = Rectangle((10,10), 100, 100)
r.fill_color = (255, 0, 0)
g = Rectangle((120,10), 100, 100)
g.fill_color = (0, 255,0)
b = Rectangle((230,10), 100, 100)
b.fill_color = (0, 0 ,255)
world.run()
A color is specified as a 3-tuple:
The first value is the red component
The second value is the green component
The third value is the blue component
By “mixing” these colors, you get a specific color:
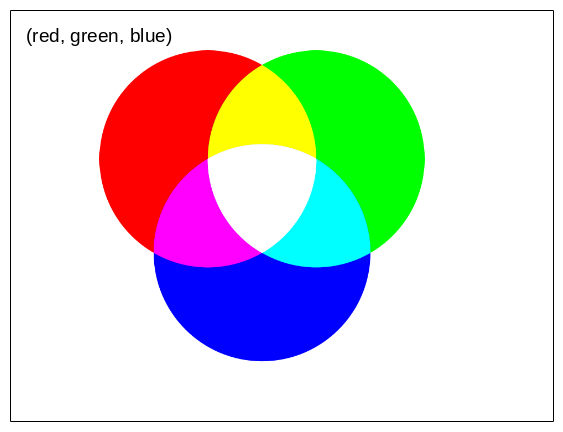
Variablen#
We have used variables here. So far, when we created an object, we could no longer access it. Here we have given the rectangles names (e.g. r) through which you can later access the objects again.
So bedeutet r.fill_color = (255, 0, 0)
, dass wir die Füllfarbe des zuvor mit r benannten Rechtecks ändern.
Border#
Jede geometrische Form kann einen Rand haben.
Den Rand kannst du als Integer-Wert mit dem Attribut border
festlegen und die Farbe mit dem Attribut border-radius
:
The following image creates a red rectangle with a yellow border:
from miniworlds import *
world = World(350, 150)
r = Rectangle((10,10), 100, 100)
r.fill_color = (255, 0, 0)
r.border = 3
r.border_color = (255, 255,0)
world.run()
Output:
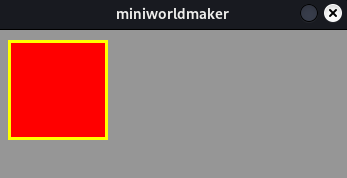
Füllung#
You can also display figures that only have an outline but no fill. The attribute fill
determines whether an object has a fill.
The following rectangle, for example, has no fill:
from miniworlds import *
world = World(350, 150)
r = Rectangle((10,10), 100, 100)
r.fill = False
r.border = 3
r.border_color = (255, 255,0)
world.run()
Die Welt#
All figures are drawn on a “World”. The World also has various properties that can be changed, such as size and background color.
Beachte folgenden Code, welcher die Größe und den Hintergrund der Welt festlegt.
from miniworlds import *
world = World()
world.add_background((255,255,255))
world.size = (400,200)
r = Rectangle((10,10), 100, 100)
r.fill = False
r.border = 3
r.border_color = (255, 255,0)
world.run()
Schulung
Übung 2.1: Black Face
Draw the following shape:
Lösungsansatz
from miniworlds import *
world = World()
world.size = (120,210)
Rectangle((10,100), 100, 100)
Triangle((10,100), (60, 50), (110, 100))
world.run()