Drawing with the miniworlds - Basics#
Preface#
This tutorial is heavily inspired by the great lecture script by Prof. Dr. Michael Kipp https://michaelkipp.de/processing/, which relates to Processing.
The miniworlds port of Processing adopts some ideas and concepts from Processing, but often implements them in a slightly different way. In this tutorial, you will learn programming with miniworlds. The miniworlds has a Processing mode that is oriented towards the popular graphics programming environment.
How do you draw on a PC?#
Monitors bestehen aus einem Gitter kleinster Quadrate. Diese Quadrate sind so klein, dass sie für uns wie Punkte aussehen. Man nennt diese kleinsten Quadrate Pixel.
The pixels are arranged in a coordinate system. However, this is slightly different because usually the origin is in the upper left corner:
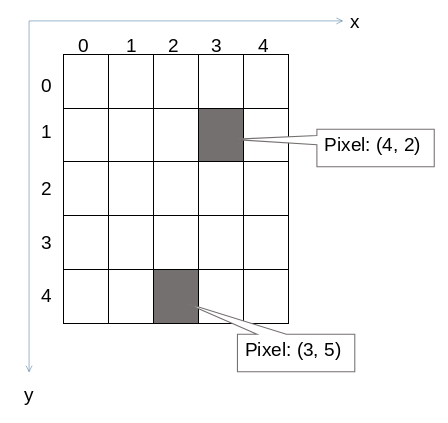
Bemerkung
Computer scientists usually start counting from 0, i.e., the top left corner has the coordinates (0,0). If the screen window is 800x600 in size, the bottom right corner has the coordinates (799, 599)
The first program#
Ein Miniworlds-Programm besteht aus mehreren Teilen:
1from miniworlds import *
2world = World()
3
4# Your code here
5
6world.run()
1: Die miniworlds-Bibliothek wird importiert
2: A playing field is being created.
6: At the end, the mainloop is started, this must always be the last line of your program.
In der Mitte befindet sich ein Kommentar - Kommentare beginnen immer mit einer # und werden vom Computer ignoriert und sind für Menschen gemacht. Sie dienen dazu, Programmierern Hinweise zu geben, hier z.B. dass an diese Stelle dein eigener Code kommt.
This could look like this, for example:
from miniworlds import *
world = World()
Point((10,10))
world.run()
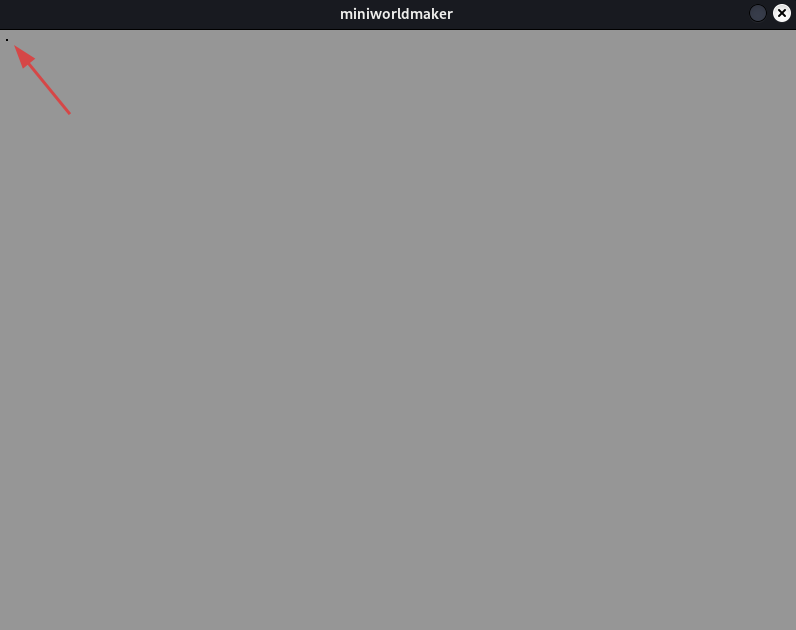
You can also adjust the size of the world by passing two arguments when creating the world:
from miniworlds import *
world = World(200, 400)
world.run()
Drawing basic geometric shapes.#
Next, you will learn to draw basic geometric shapes.
Linien#
The syntax for drawing a line is as follows:
Line(startpoint, endpoint)
The parameters startpoint
and endpoint
are each tuples, e.g., (1, 2) for x=1 and y=2.
If you want to draw a line from (10,10) to (100, 200), you must write the following, for example:
from miniworlds import *
world = World()
Line((10,10), (100, 200))
world.run()
Kreise#
You can generally create circles as follows:
Line(position, radius)
Bemerkung
The passed position in circles is the center of the circle
If you want to create a circle at the position (100,200) with a radius of 20, you must write the following:
from miniworlds import *
world = World()
Circle((100,200), 20)
world.run()
Rechteck#
A rectangle is described by position, width, and height:
Rectangle(position, width, height)
The parameter position
describes the top left corner of the rectangle.
Wenn du ein Rechteck an der Position (100, 100) mit Breite 20 und Höhe 100 zeichnen möchtest, musst du Folgendes schreiben:
from miniworlds import *
world = World()
Rectangle((100, 100), 20, 100)
world.run()
Ellipse#
Ellipsen werden im Prinzip wie Rechtecke beschrieben, d.h. die Ellipse wird dann so gezeichnet, dass sie genau in das Rechteck hineinpasst. width
und height
beziehen sich hier jeweils auf den Durchmesser der Ellipse
Ellipse(position, width, height)
To draw an ellipse at position (100, 100) with a width of 20 and a height of 100, you must write the following:
from miniworlds import *
world = World()
Ellipse((100, 100), 20, 100)
world.run()
Move rectangle and ellipse to the center.#
Oft möchte man ein Rechteck oder eine Ellipse nicht an der oberen linken Position erstellen, sondern am Mittelpunkt. Es gibt mehrere Möglichkeiten, wie man dies erreichen kann, ohne die Position manuell auszurechnen.
1. from_center#
Mit der Klassenmethode from_center kannst du eine Ellipse am Zentrum erstellen.
from miniworlds import *
world = World()
Ellipse.from_center((50, 100), 100, 200)
world.run()
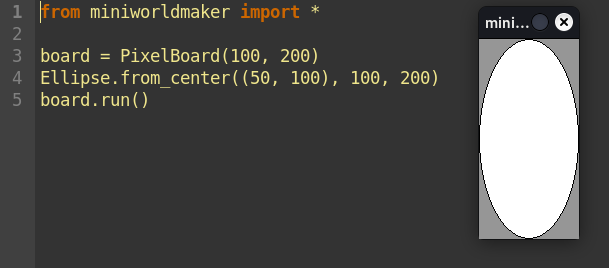
2. Verschieben mit ellipse.center#
You can move the ellipse to the center after moving it;
from miniworlds import *
world = World()
Ellipse((50, 100), 100, 200)
ellipse.center = ellipse.position
world.run()
Complex geometric basic shapes#
Bogen#
You draw circular arcs and circular segments with the following command:
Arc(position, width, height, start_angle, end_angle)
Meaning of the parameters:
position
: Die Position als 2-Tupelwidth
,height
: Breite und Höhe bei Erstellung einer Ellipse. Wähle beide gleich, damit sich der Radius für einen Kreisbogen ergibt oder wähle unterschiedliche Werte, damit sich der Bogen und Ausschnitt einer Ellipse ergibt.start_angle
,end_angle
: The angles of the segment. The angles are measured from an imaginary line counterclockwise.
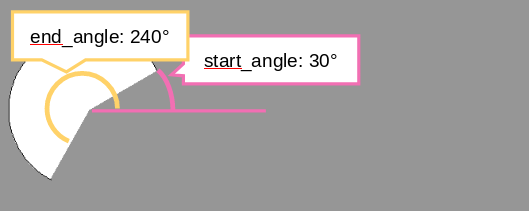
Example:#
from miniworlds import *
world = World()
a1 = Arc.from_center((200, 200), 200, 200, 30, 242)
world.run()
Dreieck#
You create a triangle with the following command:
Triangle(p1,p2, p3)
p1, p2 und p3 are points that you can write as tuples.
Example:#
from miniworlds import *
world = World()
Triangle((10,10), (100,100), (200,10))
world.run()
Vieleck#
You create a polygon (i.e., an n-gon) with the following command:
Polygon(pointlist)
pointlist
ist eine Liste von Punkten. Das Dreieck aus dem vorherigen Beispiel kannst du z.B. folgendermaßen als Polygon erstellen:
Example:#
from miniworlds import *
world = World()
Polygon([(10,10), (100,100), (200,10)])
world.run()
Schulung
Übung 1.1: Haus mit Grundformen
Zeichne ein Haus mit Grundformen:
Lösungsansatz
from miniworlds import *
world = World()
world.size = (120,210)
Rectangle((10,100), 100, 100)
Triangle((10,100), (60, 50), (110, 100))
world.run()
Übung 1.2: Gesicht
Draw a face with basic shapes
Lösungsansatz
from miniworlds import *
import random
world = World((100,100))
world.size = (100, 100)
Circle.from_center((50,50),50)
Arc.from_center((50,80),40,20, 180, 360)
Circle.from_center((30,30),10)
Circle.from_center((70,30),10)
Line((50,50),(50,70))
world.run()